So since this whole COVID-19 thing started, I’ve had a lot of free time on my hands. Yes, I’ve suddenly become a teacher (I’m taking care of my oldest, while Liz takes care of our youngest) but I am only working at the office one week out of every three. That gives me a lot of time. I decided among other things to work on my Python (a lot). So I went back to the 2015 Advent of Code and just started going away. So here are the first five days of the 2015 Advent of Code.
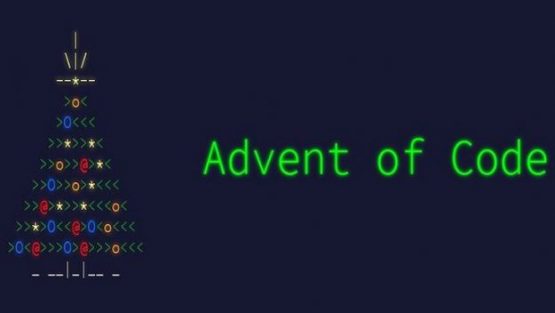
Day 1: Not Quite Lisp
This was a very easy way to kick off the year and in all honesty, I used Notepad++ and didn’t touch Python to answer this. So here is Part 1:
Santa was hoping for a white Christmas, but his weather machine’s “snow” function is powered by stars, and he’s fresh out! To save Christmas, he needs you to collect fifty stars by December 25th.
Collect stars by helping Santa solve puzzles. Two puzzles will be made available on each day in the Advent calendar; the second puzzle is unlocked when you complete the first. Each puzzle grants one star. Good luck!
Here’s an easy puzzle to warm you up.
Santa is trying to deliver presents in a large apartment building, but he can’t find the right floor – the directions he got are a little confusing. He starts on the ground floor (floor
0
) and then follows the instructions one character at a time.An opening parenthesis,
(
, means he should go up one floor, and a closing parenthesis,)
, means he should go down one floor.The apartment building is very tall, and the basement is very deep; he will never find the top or bottom floors.
For example:
(())
and()()
both result in floor0
.(((
and(()(()(
both result in floor3
.))(((((
also results in floor3
.())
and))(
both result in floor-1
(the first basement level).)))
and)())())
both result in floor-3
.To what floor do the instructions take Santa?
All I did here was copy my problem input into Notepad++ and do a Word Count of the ‘(‘ and then for the ‘)’ and then subtract the difference. Easy Peezy. Part 2 wasn’t quite as easy, but still pretty damn easy.
Now, given the same instructions, find the position of the first character that causes him to enter the basement (floor
-1
). The first character in the instructions has position1
, the second character has position2
, and so on.For example:
)
causes him to enter the basement at character position1
.()())
causes him to enter the basement at character position5
.What is the position of the character that causes Santa to first enter the basement?
This one, I just looped through the different “steps” and kept track with a counter of what floor we were on until it went below 0. You can see the solution by going to https://github.com/pyrodie18/AdventOfCode15/tree/master/Day1
Day 2: I Was Told There Would Be No Math
The elves are running low on wrapping paper, and so they need to submit an order for more. They have a list of the dimensions (length
l
, widthw
, and heighth
) of each present, and only want to order exactly as much as they need.Fortunately, every present is a box (a perfect right rectangular prism), which makes calculating the required wrapping paper for each gift a little easier: find the surface area of the box, which is
2*l*w + 2*w*h + 2*h*l
. The elves also need a little extra paper for each present: the area of the smallest side.For example:
- A present with dimensions
2x3x4
requires2*6 + 2*12 + 2*8 = 52
square feet of wrapping paper plus6
square feet of slack, for a total of58
square feet.- A present with dimensions
1x1x10
requires2*1 + 2*10 + 2*10 = 42
square feet of wrapping paper plus1
square foot of slack, for a total of43
square feet.
Once again, I cheated. I put together a simple Excel spreadsheet that did all of the math for me. I wish I had saved a copy of it, but I don’t think I did. Once again though, Part 2 wasn’t as easy and actually needed a tiny bit of Python.
The elves are also running low on ribbon. Ribbon is all the same width, so they only have to worry about the length they need to order, which they would again like to be exact.
The ribbon required to wrap a present is the shortest distance around its sides, or the smallest perimeter of any one face. Each present also requires a bow made out of ribbon as well; the feet of ribbon required for the perfect bow is equal to the cubic feet of volume of the present. Don’t ask how they tie the bow, though; they’ll never tell.
For example:
- A present with dimensions
2x3x4
requires2+2+3+3 = 10
feet of ribbon to wrap the present plus2*3*4 = 24
feet of ribbon for the bow, for a total of34
feet.- A present with dimensions
1x1x10
requires1+1+1+1 = 4
feet of ribbon to wrap the present plus1*1*10 = 10
feet of ribbon for the bow, for a total of14
feet.How many total feet of ribbon should they order?
This one required that I find the smallest two sides to figure out the extra ribbon. I did this breaking each set of dimensions into a list, and then sorting the list so that the smallest sides were always in the same location within the list so the math was pretty easy at that point. You can find my solution at https://github.com/pyrodie18/AdventOfCode15/tree/master/Day2.
Day 3: Perfectly Spherical Houses in a Vacuum
Santa is delivering presents to an infinite two-dimensional grid of houses.
He begins by delivering a present to the house at his starting location, and then an elf at the North Pole calls him via radio and tells him where to move next. Moves are always exactly one house to the north (
^
), south (v
), east (>
), or west (<
). After each move, he delivers another present to the house at his new location.However, the elf back at the north pole has had a little too much eggnog, and so his directions are a little off, and Santa ends up visiting some houses more than once. How many houses receive at least one present?
For example:
>
delivers presents to2
houses: one at the starting location, and one to the east.^>v<
delivers presents to4
houses in a square, including twice to the house at his starting/ending location.^v^v^v^v^v
delivers a bunch of presents to some very lucky children at only2
houses.
For this one, I started out by making a grid of “houses”. I tried a few different sizes but ended up a grid 250 x 250 was a good size, and then I plopped Santa down in the middle of it. From there, I looped through each instruction and decoded it. When I got to the next house, I would look and see what value it had (all houses started with 0). If it was still 0, I incremented my Total Houses counter and then incremented the counter for that house (I figured it would probably be useful for part two). If the house counter was already greater than 0, than I just incremented it again, but didn’t increase the Total Houses counter.
The next year, to speed up the process, Santa creates a robot version of himself, Robo-Santa, to deliver presents with him.
Santa and Robo-Santa start at the same location (delivering two presents to the same starting house), then take turns moving based on instructions from the elf, who is eggnoggedly reading from the same script as the previous year.
This year, how many houses receive at least one present?
For example:
^v
delivers presents to3
houses, because Santa goes north, and then Robo-Santa goes south.^>v<
now delivers presents to3
houses, and Santa and Robo-Santa end up back where they started.^v^v^v^v^v
now delivers presents to11
houses, with Santa going one direction and Robo-Santa going the other.
The second part required only a few minor changes to my code. I essentially just created a second set of variables to track where the Robot was separate from Santa and then switched back and forth between who was moving based on if the instruction count was even or odd. Turned out that I didn’t need to track the number of times I visited a house (oh well) but everything else was the same. Both parts of the solution can be found at https://github.com/pyrodie18/AdventOfCode15/tree/master/Day3.
Day 4: The Ideal Stocking Stuffer
Santa needs help mining some AdventCoins (very similar to bitcoins) to use as gifts for all the economically forward-thinking little girls and boys.
To do this, he needs to find MD5 hashes which, in hexadecimal, start with at least five zeroes. The input to the MD5 hash is some secret key (your puzzle input, given below) followed by a number in decimal. To mine AdventCoins, you must find Santa the lowest positive number (no leading zeroes:
1
,2
,3
, …) that produces such a hash.For example:
- If your secret key is
abcdef
, the answer is609043
, because the MD5 hash ofabcdef609043
starts with five zeroes (000001dbbfa...
), and it is the lowest such number to do so.- If your secret key is
pqrstuv
, the lowest number it combines with to make an MD5 hash starting with five zeroes is1048970
; that is, the MD5 hash ofpqrstuv1048970
looks like000006136ef...
.
This one took more time to understand exactly what they were saying than actually solve it. Basically they give you the problem input, and then you need to find an integer (as a string) that you can append to it to create a hash that has a bunch of leading zeros. The only real way to do this is just brute force it, which is exactly what I did. The 2nd part was exactly the same, except the added one more leading zero. The solution can be found at https://github.com/pyrodie18/AdventOfCode15/tree/master/Day4.
Day 5: Doesn’t He Have Intern-Elves For This?
Santa needs help figuring out which strings in his text file are naughty or nice.
A nice string is one with all of the following properties:
- It contains at least three vowels (
aeiou
only), likeaei
,xazegov
, oraeiouaeiouaeiou
.- It contains at least one letter that appears twice in a row, like
xx
,abcdde
(dd
), oraabbccdd
(aa
,bb
,cc
, ordd
).- It does not contain the strings
ab
,cd
,pq
, orxy
, even if they are part of one of the other requirements.For example:
ugknbfddgicrmopn
is nice because it has at least three vowels (u...i...o...
), a double letter (...dd...
), and none of the disallowed substrings.aaa
is nice because it has at least three vowels and a double letter, even though the letters used by different rules overlap.jchzalrnumimnmhp
is naughty because it has no double letter.haegwjzuvuyypxyu
is naughty because it contains the stringxy
.dvszwmarrgswjxmb
is naughty because it contains only one vowel.How many strings are nice?
To solve this one, I first created a few lists that included all of the vowels as well as all of the bad pairs. Then it loops through each input line and checks for the total number of vowels being greater than three, that it doesn’t find any naught pairs, cycles through all of the letters in the string looking for a pair (string[x] == string[x+1]).
Realizing the error of his ways, Santa has switched to a better model of determining whether a string is naughty or nice. None of the old rules apply, as they are all clearly ridiculous.
Now, a nice string is one with all of the following properties:
- It contains a pair of any two letters that appears at least twice in the string without overlapping, like
xyxy
(xy
) oraabcdefgaa
(aa
), but not likeaaa
(aa
, but it overlaps).- It contains at least one letter which repeats with exactly one letter between them, like
xyx
,abcdefeghi
(efe
), or evenaaa
.For example:
qjhvhtzxzqqjkmpb
is nice because is has a pair that appears twice (qj
) and a letter that repeats with exactly one letter between them (zxz
).xxyxx
is nice because it has a pair that appears twice and a letter that repeats with one between, even though the letters used by each rule overlap.uurcxstgmygtbstg
is naughty because it has a pair (tg
) but no repeat with a single letter between them.ieodomkazucvgmuy
is naughty because it has a repeating letter with one between (odo
), but no pair that appears twice.How many strings are nice under these new rules?
The 2nd part took a minute to understand because the “naughty” conditions are a little bit odd. In the end, it was a minor modification to look for repeating letters, and then a little bit of creative thinking to figure out how to find the repeating pairs. This part here especially took some debugging to get it working because it would tag correctly on some pairs, but not on others. Getting the logic just right so I wouldn’t get an out of bounds error while still checking them all was kinda a pain.
Other Thoughts
As I write this, I am already just about done with most of 2015, but I figured posting 5 at a time was enough. On top of that I have also been studying to get my CCNA back alive.